Easier Block list
- Wilfre
- 8 avr. 2018
- 3 min de lecture
This is the old block list :
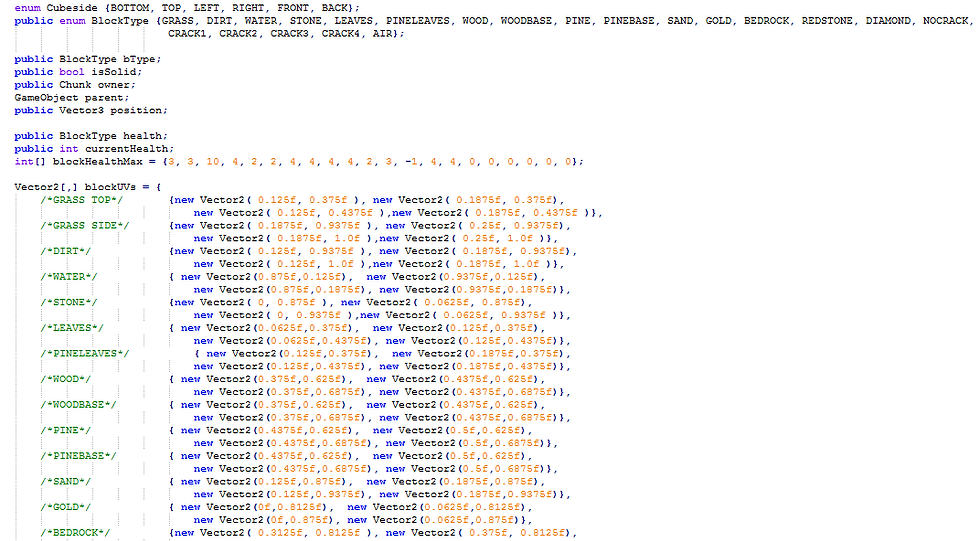
But having those numbers may be too much to work with and you may want to quickly add new blocks to your project.
But what should i do?
First, you will have to understand how the list works.
We use a Vector2 to store the texture position in the atlas. It gives an X,Y position to the texture and an easy way to store it.
Each texture position in the texture atlas is in the blockUV array with its index corresponding to the index of a block in the BlockType enum (Keep in mind that an enum is a list of choices)
Here, the texture positions are calculated and you added them to the array.
But why not let Unity find those values?
How are the textures calculated?
Let me show you with a 4x4 texture atlas :
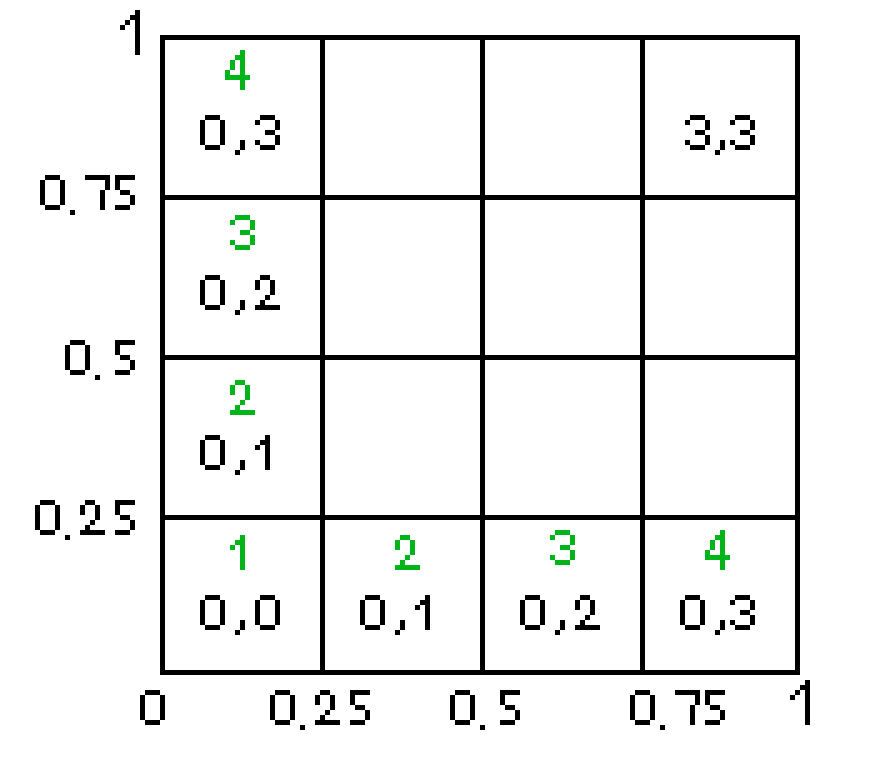
What we need is one texture in the 4x4 atlas, the texture offset is 1/4, giving the size of one texture in the atlas.
Let's use the 0,0 :
This is how UVs are defined in the course
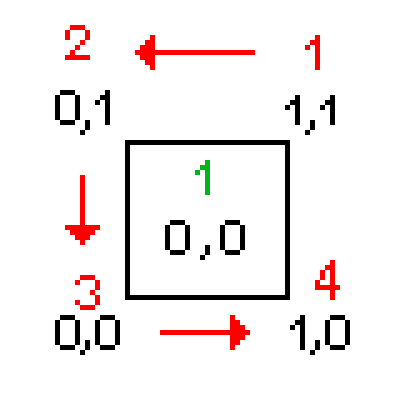
We start by giving the 1,1 coordinates and then
0,1
0,0
1,0
So, to calculate the coordinates, let's use the offset we had, 1/4 (One texture on a 4x4)
1,1 will be : (1/4 = 0.25 and 1/4 = 0.25)
0,1 : (0/4 = 0 and 1/4 = 0.25)
0,0 : (0/4 = 0 and 0/4 = 0)
1,0 : (1/4 = 0.25 and 0/4 = 0)
Giving the values :
1,1 = new Vector2(0.25, 0.25)
0,1 = new Vector2(0, 0.25)
0,0 = new Vector2(0, 0)
1,0 = new Vector2(0.25, 0)
Now that we know how to calculate the values, we will let Unity do it automatically :
In the course, to set the UVs, when we called the "CreateQuad" function to create a quad of the cube, we had to check which texture has to be used.
It works like that :
Create 4 UV possibilities (4 vertices/corners) :
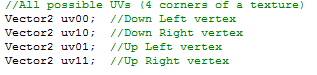
Then, we store the texture we will use for the new quad inside the variables we just created :
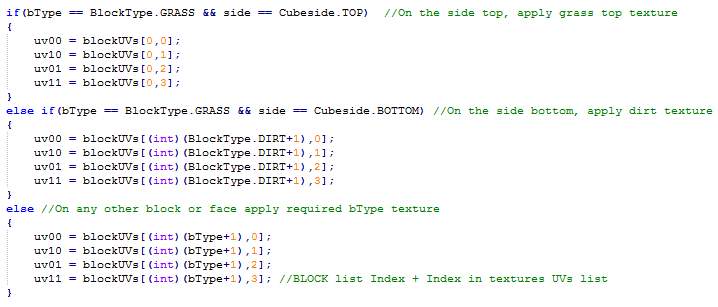
Let me explain.
To apply the corresponding texture to the quad, we will check which block it is. When this method is called, when a quad is created, we already ask for a block type and give the requested side of the cube it will be. From now, we play with conditions. Having multiple textures for a block or full blocks.
Here,
- If it is a Grass block and we are creating the Top side, we apply the Grass Top texture we added in the blockUVs array (With all those numbers). We set it with the index of the texture stored in the array.
- If it is the left/right/front/back, we use the Grass Side texture.
- If it is none of them or another block, we just use the texture index corresponding to the selected block.
But all those textures stored in the blockUVs array are given manually. That's where the fix is used.
First, we will remove the blockUVs array and the conditions

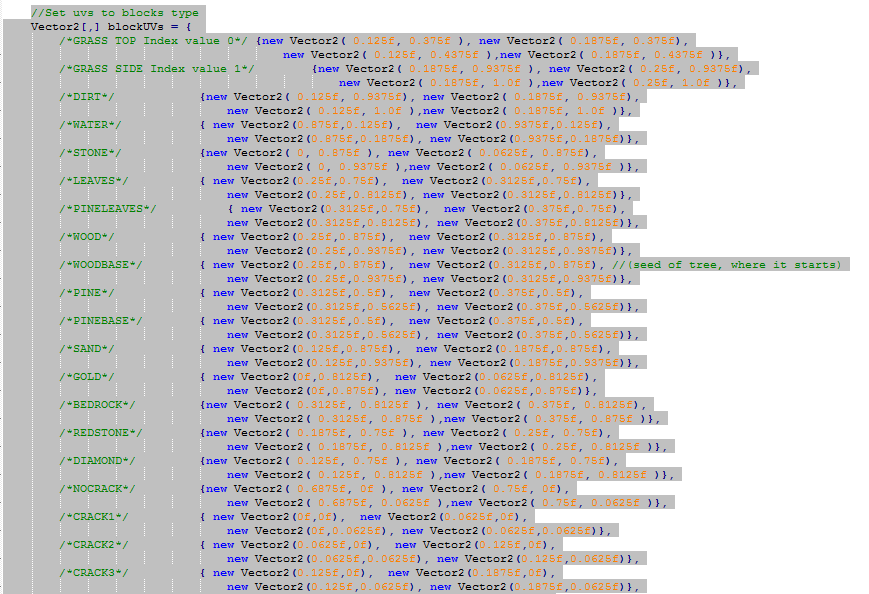
And now the fix :
I will use this 4x4 texture atlas
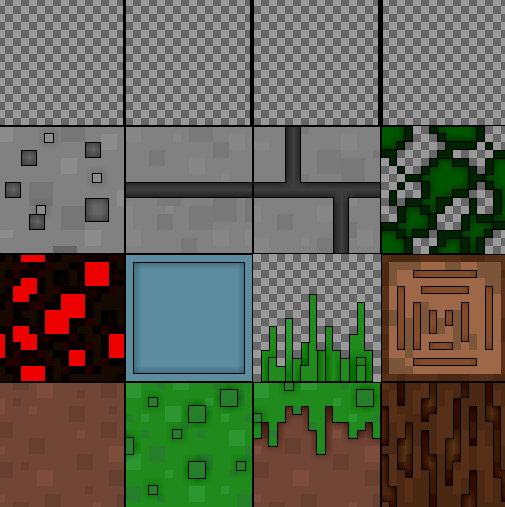
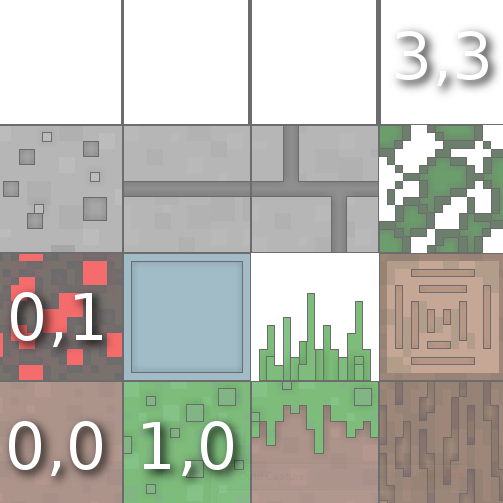
Then we will create a method to get the texture we need. It will be returned in a Vector2.
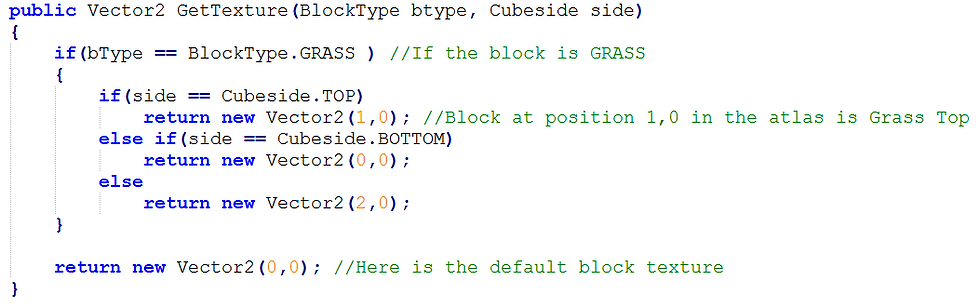
To explain :
public Vector2 GetTexture(BlockType btype, Cubeside side)
When called, this method will give a Vector2. We give the block and which side of the block and it gives back the corresponding texture.
if(bType == BlockType.GRASS )
If the block called is "Grass" from the enum "BlockType"
if(side == Cubeside.TOP)
In this block, if the side we are looking at is TOP
return new Vector2(1,0);
We answer back "The texture in the atlas, for the top side of the grass block is at 1,0", Vector2(1,0)
After adding every block possibilities, we have a last line :
return new Vector2(0,0);
This is the default texture used if none of the requirements are in the conditions. If the side doesn't exist or the block called doesn't exist.
Finally, we call the method automatically :
We replace :
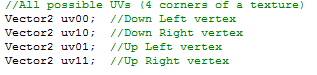
With :
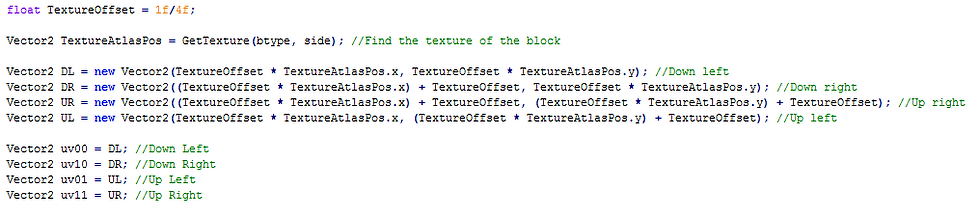
To explain :
float TextureOffset = 1f/4f;
Like i said earlier, the we tell Unity the texture offset, 1 texture in a 4x4 atlas.
Vector2 TextureAtlasPos = GetTexture(btype, side);
Then we find and store the texture of the quad we are creating in a variable.
Vector2 UL = new Vector2(TextureOffset * TextureAtlasPos.x, (TextureOffset * TextureAtlasPos.y) + TextureOffset);
Exactly like i did earlier :
1,1 = 1/4 = 0.25 * number of textures on x, (0.25 * nb of textures on y) + 1/4 on y to have the up left vertex
Vector2 uv01 = UL;
And then we store it and the code already existing will take care of the rest.
Comentarios