This time we will start working on the new project. And we will first create the block database. So we will have something to work with through the course.
We will use Json, so make sure you check the guide about it here
Open Unity and create your new project.
We will need the special folder called "StreamingAssets" which i explained in my Xml/Json guide. It allows us tu add and edit files that belongs to the computer and are not changed by Unity.
We also need a new text file in it called "blocks.json" (which i decided to put in a "database" folder). I also decided to avoid uppercase to keep a way to recognize special folders which i access instead of using them for file management.

(The file icon is showing what program you selected to open Json files. For me it is "Brackets". But any text editor can open it).
Now let's open the file and add some values to it. What we need is a block. Just one for now.
We will create the structure with "[ { } ]" and put the values of it. I want to make sure the block has every value it needs. If you forget one, you will have to add it to every other block. Usually, programmers create tools that accesses json elements and edit them so they don't have to do it manually. But here i won't, so it's better to think of everything before.
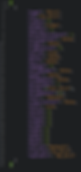
Name : The title of the block.
Id : The unique name of the block
Index : The unique number of the block
Shape : Cube, mesh, billboard, ...
Health : Number of hits for a normal attack
BlastResistance : For explosions resistance
Transparent : Can see through the block?
Light : Emits light around it?
LightForce : How far does it emit light?
Solid : Can we collide with the block?
Water : Can the block flow like water/lava?
Hurt : Does the block hurt the player?
Damage: How much does it hurt?
AllowPower: Can power go through it?
Power: Does it create power?
Flammable: Does it burn?
DropIndex: Number of drops it has in list
Drops: List of items it can drop (IDs)
DropAmount: How much of each item
Tool: Which tool type is better to break?
RequiresTool: The player must have tool?
Full: Is the texture on every side?
FullX,FullY: Position of the texture in atlas
SideX, SideY: Position of sides if not full
TopX, TopY: Position ot top texture
BotX, BotY: Position of bottom texture
TxFile: Which texture file to use if multiple
IcoFile: What is the filename of it's icon
AudioKit: What group of sounds is it in?
Here are all the values the block needs for now. Most of them may even not be used in this course.
Also notice that editing Json files in this folder doesn't need compilation as it is accessed via the scripts.
Now let's generate the Database in Unity!
Go back to Unity and this time, create a new folder called "Scripts" and another one called "Databases" inside it. Then create a script called "DB_Blocks".
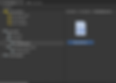
In this course i will use a lot of prefixes for variables and names. They all have a meaning.
nt_dirt : Nature type block _ dirt
nti_dirt : Nature type Item _ dirt
ak_dirt : AudioKit _ dirt
Db_Blocks : Database type script _ Blocks
Open the script and let's start writting it.
The first thing we need to do is remove the MonoBehaviour reference to only have a basic class and the Start and Update methods. Also, we make the class static so there is only one instance of this class existing. We don't need another block database.
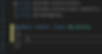
Now we will also create the Block class which defines one block and it's values.
We add it in the same script, under the database class.
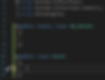
One of the longest parts comes here, this is where we will create variables for each data of the block we have, with default values for an empty block :

They are all the ones you have in the Json file. They will be stored here.
I also use regions to separate parts and close them to make the code more readable.
Under the variables we can add two constructors. These will be the default block, the "Air" block. And any other block which we create.
In the main constructor, i will only ask for the values i need for now, i don't need more for a few guides.

Notice that i use an empty prefix, such as "_name". It means that the variable is local, only for this constructor. It is not really important but i like to keep track of local variables and their range.
Finally, let's add some methods to return the block values.
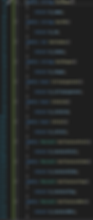
When they are called, they give back the block data we need. It will avoid checking for each one, we ask the block if it is transparent instead of checking if it is air, or leaves, or glass, ...
Now let's go back to the "DB_Blocks" class and create the database!

We need a list, called "blocks" which will contain every block in the game. And a method called "GenerateDatabase" used to find and store blocks in the database to make them exist in the memory.
Now we want to read the Json file. And just like the other guide, we need a library called "LitJson".
Then create a new folder in Unity called "Plugins" and put the .DLL file inside it.

Don't worry if there is no icon, it is because you didn't choose a program to open it.
Now we can import it in our script :

(Again, this is explained in the Xml/Json guide)
Let's get the elements from the file. We need to store the data in a JsonData object and map the text to it.

Make sure you are using System.IO to access the text and read it
We can now build each block from the Json file by getting their values. (Here only one block).
They are used in the constructor and then the new block is stored in the database.

Every value needed to construct the block must be given.
Now, what if we want to find a block in the database?
Let's add a method called "GetBlock", this will ask for an ID, to give the unique block with the same ID.

We look at each block in the database. And if one of them has the same id as the one required (using "GetID" we added to the block), we give it back to the caller and stop.
If we can't find it, we give back a new Block, which is an Air block or a "null" block with default values.
The game world is actually filled with air blocks in empty spaces, so we still have blocks and avoid errors if we don't find anything. Having a null value is extremely bad.