Implementing XML and Json files
- Wilfre
- 23 déc. 2018
- 6 min de lecture
What if you'd like to let the players mod the game or have an easy access to new blocks and their values? Let's do it here!
What are XML and Json files?
XML (Extensible Markup Language) and Json (JavaScript Object Notation) files are usually used for data management. We will use a XML file to store folders and configuration values and let Unity read the values stored in it. And the Json file will list each block and each values of them inside. This way we can let players or yourself add more and more anytime you want. Because these files are accessed from your folders instead of Unity, your data won't be deleted when you edit your scripts.
Here are examples :
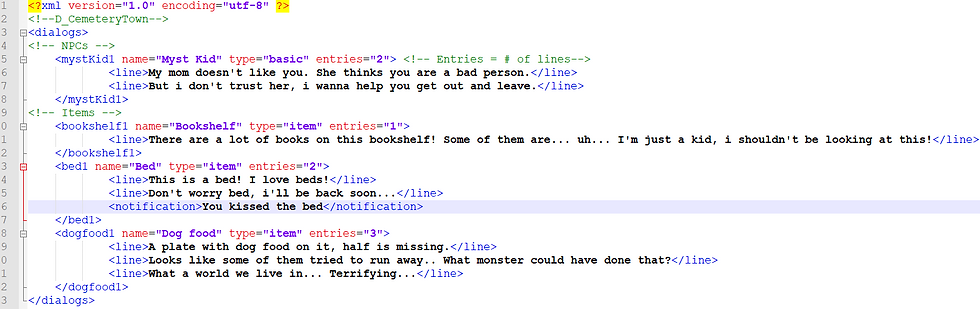
This is a XML file containing dialogs and events in a game. We tell Unity which NPC or Object is "talking" and it will look in this file for every line before using them.
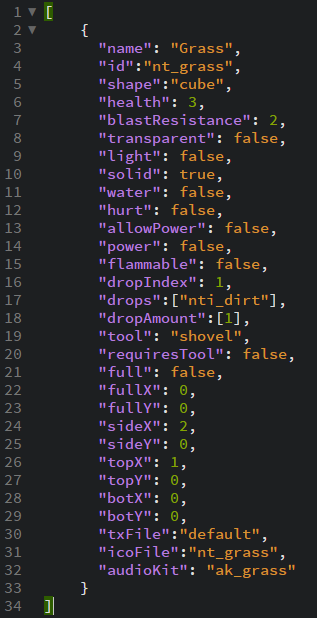
This one is a Json file which contains one block. There is a list of each of it's values which will be read by Unity. Then the block will be stored in the game local database to be called and read at any time.
Let's make it work in Unity!
First, the XML files. This time we will create a configuration file for our game.
We need values to get called on the game creation. This file can be accessed by the players and edited. To do that, we will create a folder in Unity called "StreamingAssets".
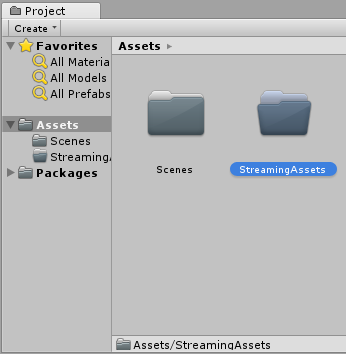
This folder is a "Special folder" which is not built during the project generation process. Instead, it is copied. So the files appears for the user and can be added or changed. This is useful to add Texture packs or mod the game.
This time we will open the folder in the Explorer and inside it, we create a new text file called "config.xml".
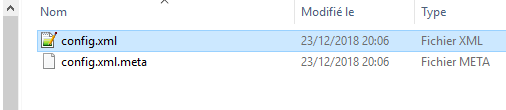
This is the file that Unity will read. Also don't delete the .meta file. It is created by Unity to store the editor settings applied to the file but won't be added to the game build.
Now open your file and let's add a few lines :

<?xml version="1.0" encoding="utf-8" ?>
This is not always used but tells the reader how to handle the file.
<config></config>
Those tags are the "main" tags. They contain every section of our XML file. They are the body or the main container. You can give it any name you want
(Note that they are written just like in HTML. But here you give your own titles to the tags)
<gametitle>UDVM</gametitle>
<playernb>4</playernb>
These are elements of the file. Each one has a unique title which you decide and the value between each part of the tag will be what Unity reads. Here the game title and the number of players.
Now go back to Unity and this time, create a new folder called "Scripts" and a script called "GameManager" in it.

You will notice that Unity changes the icon because the name is commonly used by developers and makes it easier to recognize the file as an important one. It will be treated as a normal script file but this is the most important script. It creates and manages the whole game and i recommend to always have one in your projects to store global values and methods.
Let's edit it in our IDE.
First we need variables for each value in the config file, and only keep the "Start" method.
We will also create another method called "LoadConfig" which is requested in the Start method.
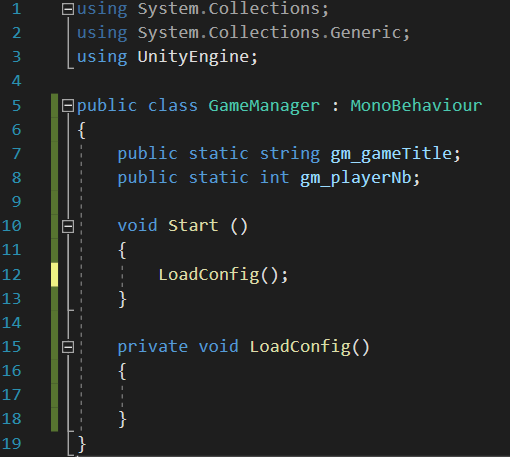
Variables are static, which means they won't change for every instance of GameManager. Also, we don't need to reference an instance but only the script to get the values. Which is faster and easier.
The LoadConfig method is private to make sure only this script will be able to call it.
Let's read our XML file. First we need to get it and store it as a text. We will put it in a string.
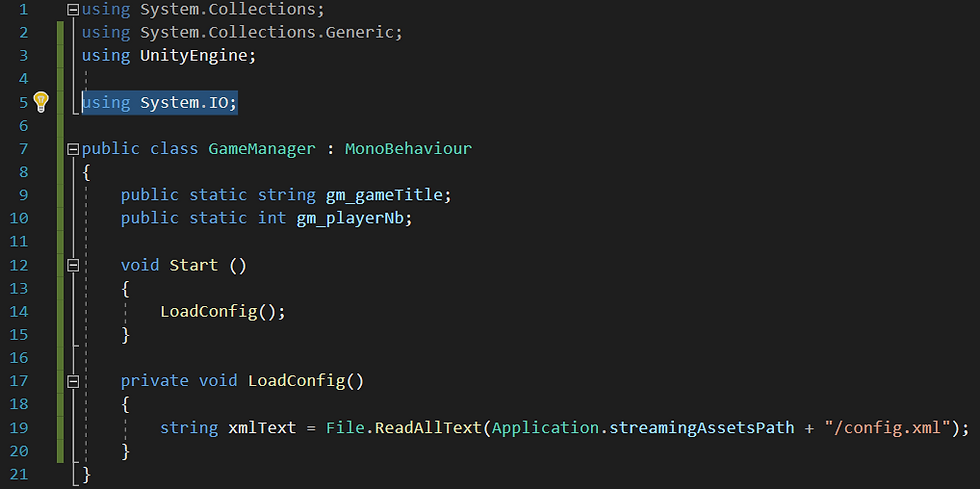
The "File" class is from the "IO" namespace (In/Out) which is used to access the computer files. Make sure you call the "System.IO" namespace. Then we use it's method called "ReadAllText" which is similar to taking any file and opening it with a text editor but here we store everything in a string. We give it a path, which starts from "Application.streamingAssetsPath", giving the "StreamingAssets" folder path for the actual game, and from it we access the "config.xml" file using "/" to navigate to the next file or folder.
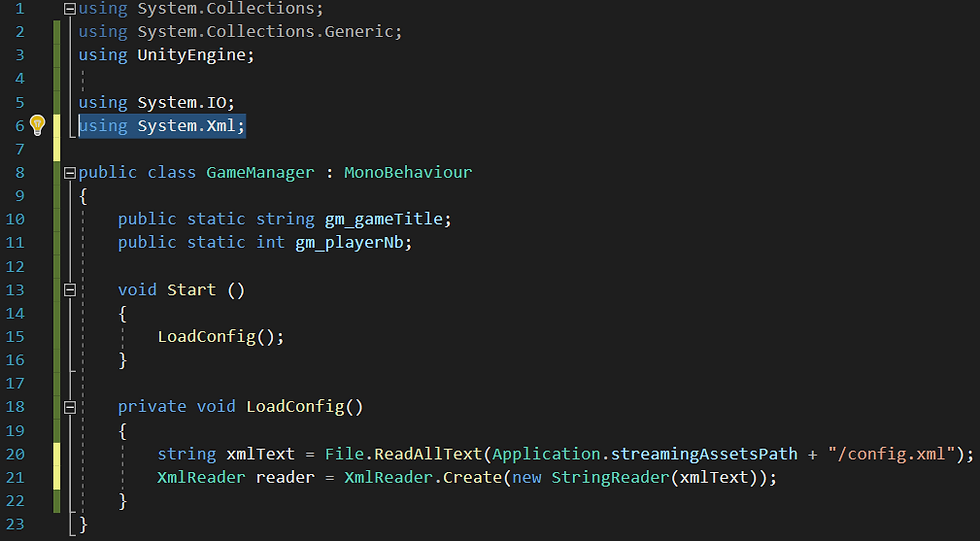
We continue by adding a "XmlReader" which is an object that can decrypt the XML file and our elements. We need the Xml namespace to add it to the script.
We create a new XmlReader by reading the string of xmlText.
Now we make it read the text and every time we find something interesting we stop and use it :
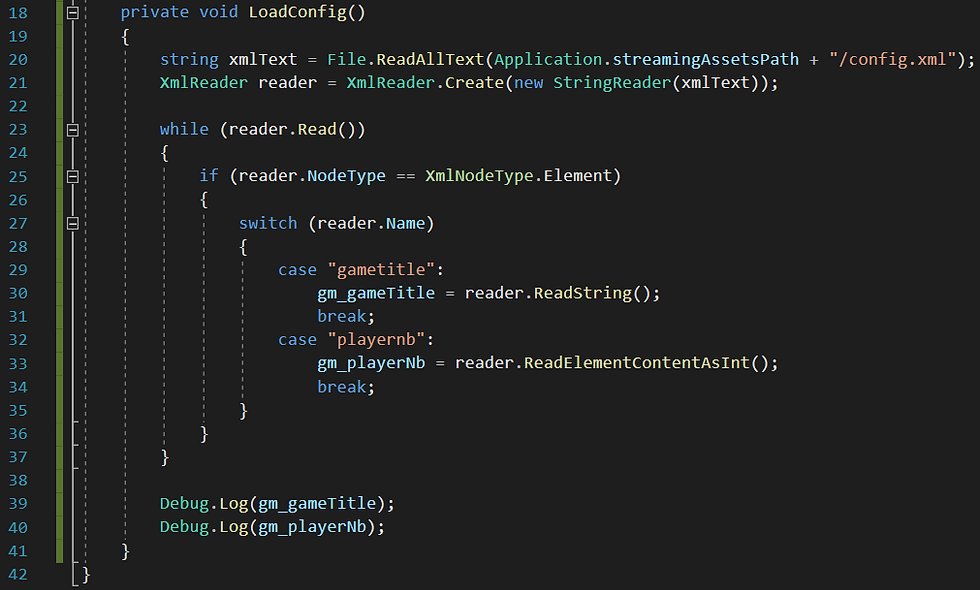
Here we ask the reader to read the file, and while it is doing it, if we find an "element", a node (which i called "tag") which is of type "element", we check what it is. And for each case we tell what to do with it. If it's name is "gametitle", we store it as a string in 'gm_gametitle' and if it is called "playernb", we store in as an int in 'gm_playerNb'. Then, when it is done reading, we display the values we found.
Now go back to Unity and create an object called "GameManager", which contains the script. And test the game.
Look at the console :
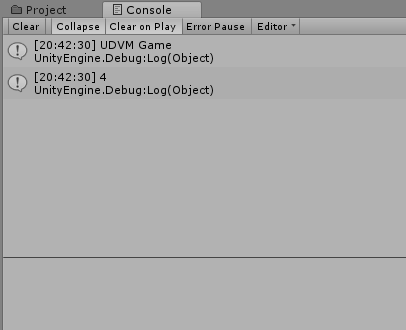
Unity managed to find the config values and to show them. Now the user is able to access them and edit them.
(If you want to limit the access, you can store the file in the "resources" folder which is then built in Unity. Then read the text file as a "TextAsset" when loaded. This won't be a topic for this guide).
Now let's read a Json file!
You'll need a library called "LitJson" which has every method to read and write Json files from your C# projects easily.
Go to Unity and create a new folder, called "Plugins". Add the LitJson.dll file inside.
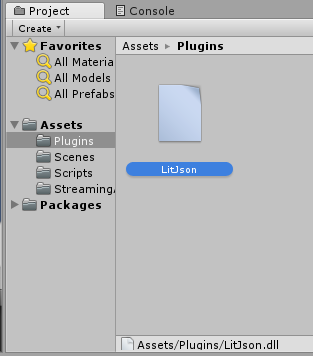
This folder is also a Special folder which takes libraries that you want to add to Unity (.dll files)
Now you can go back to your script.
Add the new namespace to the list to use the LitJson library.

Now Unity can read Json files. But we didn't make one. Go to the folder where you created the XML file and make a new text file called "config.json". Open it with a text editor and write this :
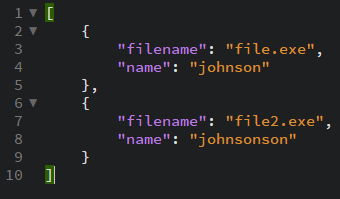
Let's understand how it works :
"[" ... "]"
Here, brackets are used to let the reader know where is the code. The beginning and the end of it.
"{" ... "}"
The Curly brackets here are used the same way but to define one element in the list. We use a comma to separate two elements.
"filename": "file.exe",
"name": "johnson"
These are the date of the elements. They give values for each one. In this example, it could be a file called "file.exe" that belongs to "johnson". And the other element is a file called "file2.exe" that belongs to "johnsonson". But there are just data, whthout a logic. When an application reads it, it creates the logic behind the values. Let's do it!
Go back to your script in Unity. After the config from the xml file is loaded, we can call the Json reader method called "LoadJsonConfig".
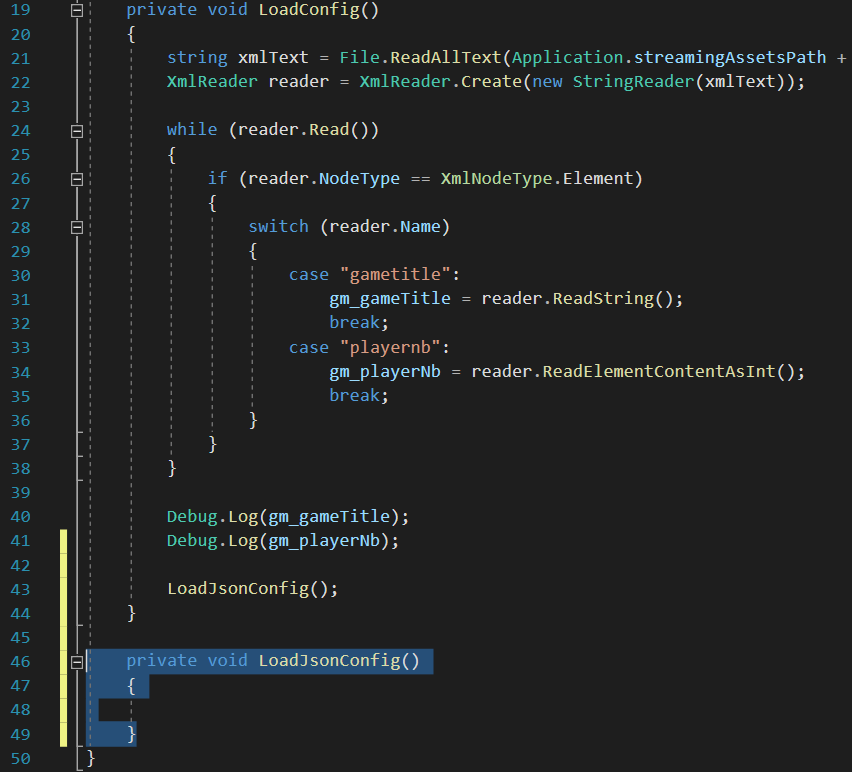
We can now start reading our values from the file.
Add a few lines :

The "JsonData" is an object containing the elements and values of our Json file.
We use a class called "JsonMapper" which converts data to text in our Json file or to an Object with values in the JsonData object.
We do the same as with the XML, we convert to an object from the text in the file. Going into the StreamingAssets folder and the "config.json" file.
Now the Json file is converted to data. We can take it out and print it :
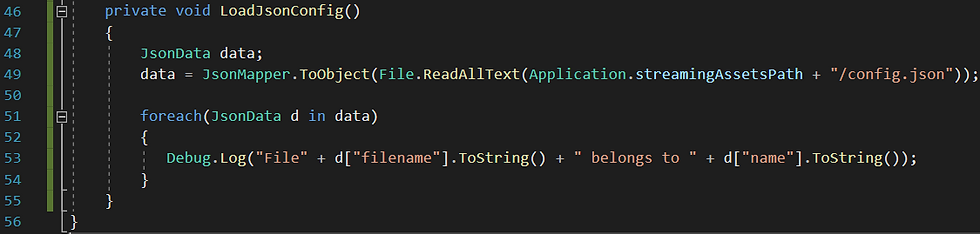
We read each JsonData element in the data object, which we store in d each time we look at one. And then access the data using :
d["variable"].ToString()
Or we can convert it to an int if a number is needed :
(int)d["variable"]
Now go back to Unity and test your project again. Looking at the console.
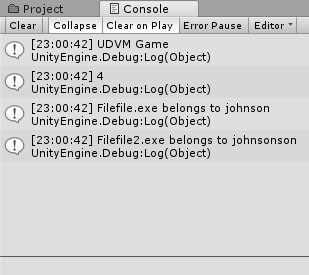
Both of the files are read, in the correct order.
Now you know how to read XML and Json files in Unity.
Comments